Published: October 30, 2020
A List of Every Named-CSS Colour
I created a small page to display every named-css colour (or color, depending on your flavour (or flavor!) of English)!)
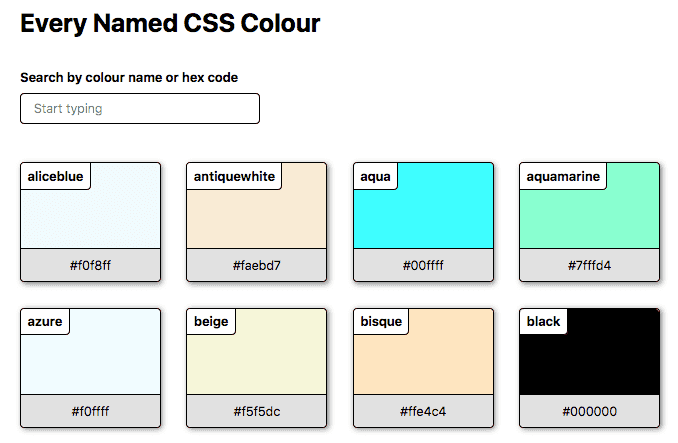
Bahamas10 has a very handy list of each named-css colour in JSON format.
With a small amount of JavaScript, you can grab that list, and interrogate it to display the name and hex code of each item, like so:
const baseURL = 'https://raw.githubusercontent.com/bahamas10/css-color-names/master/css-color-names.json';
const list = document.querySelector('.colour-list');
const input = document.querySelector('.colour-search__input');
fetch(baseURL)
.then(resp => resp.json())
.then(data => {
Object.keys(data).forEach(datum => {
listItem = document.createElement('li');
listItem.classList.add('list-item');
listItem.innerHTML = `
<div class="list-item__panel" style="background-color: ${data[datum]}">
<span class="list-item__name">${datum}</span>
</div>
<div class="list-item__hex">${data[datum]}</div>
`
list.appendChild(listItem);
});
const listOfColours = document.querySelectorAll('.list-item');
function handleInputChange() {
let inputValue = input.value.toLowerCase();
listOfColours.forEach(listOfColour => {
if (!listOfColour.innerText.toLowerCase().includes(inputValue)) {
listOfColour.style.display = 'none';
} else {
listOfColour.style.display = 'flex';
}
});
}
input.addEventListener('input', handleInputChange);
});
And then pop that inside some HTML that looks like this:
<div class="colour-search">
<label class="colour-search__label" for="search">Search by colour name or hex code</label>
<input class="colour-search__input" placeholder="Start typing" type="text" id="search">
</div>
<ul class="colour-list"></ul>
Which will then give you a nice input selector to search for any colour by name or hex code.
You can view the page here or checkout the code on GitHub. Enjoy.